ID |
Date |
Author |
Author Email |
Category |
Subject |
Status |
Last Revision |
148
|
Mon Mar 11 09:28:15 2019 |
Maurat | gm001@free.fr | Script | Code change for LDAP authentication | Stable | Mon Mar 11 10:15:43 2019 by Maurat | Hi,
I had to change code to authenticate users in my organization's LDAP directory. Indeed, accounts are distributed under several organizational units in my LDAP directory.
The current version of the code can't authenticate accounts when these are in different organizational units. Hence my contribution.
I Use a read account to request LDAP to locate the account that has logged in (with e-mail address in the search filter).
I get the number of LDAP entries. If I have one entry then I call ldap_get_dn function to get the DN account and then I call ldap_simple_bind_s using the account's DN and password to perform LDAP authentication.
I changed configuration file elogd.cfg. I added two parameters:
LDAP DN user = <DN read account>
LDAP PW user = <password read account>
I changed code auth.c too (see attached file)
I had to change Makefile. I added a call to lber library
ifdef USE_LDAP
ifneq ($(USE_LDAP), 0 )
CFLAGS += -DHAVE_LDAP
LIBS += -lldap -llber
endif
endif
Have good day
|
Attachment 1: auth.c
|
/********************************************************************\
Name: auth.c
Created by: Stefan Ritt
Copyright 2000 + Stefan Ritt
ELOG is free software: you can redistribute it and/or modify
it under the terms of the GNU General Public License as published by
the Free Software Foundation, either version 3 of the License, or
(at your option) any later version.
ELOG is distributed in the hope that it will be useful,
but WITHOUT ANY WARRANTY; without even the implied warranty of
MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
GNU General Public License for more details.
You should have received a copy of the GNU General Public License
along with ELOG. If not, see <http://www.gnu.org/licenses/>.
Contents: Authentication subroutines. Currently supported:
- password file authentication
- kerberos5 password authentication
$Id: elog.c 2350 2010-12-23 10:45:10Z ritt $
\********************************************************************/
#include "elogd.h"
#ifdef HAVE_KRB5
#include <krb5.h>
#endif
#ifdef HAVE_LDAP
#include <ldap.h>
LDAP *ldap_ld;
char ldap_login_attr[64];
char ldap_dn_user[256];
char ldap_pw_user[64];
char ldap_userbase[256];
char ldap_bindDN[512];
#endif /* HAVE_LDAP */
extern LOGBOOK *lb_list;
/*==================================================================*/
/*---- Kerberos5 routines ------------------------------------------*/
#ifdef HAVE_KRB5
int auth_verify_password_krb5(LOGBOOK * lbs, const char *user, const char *password, char *error_str,
int error_size)
{
char *princ_name, str[256], realm[256];
krb5_error_code error;
krb5_principal princ;
krb5_context context;
krb5_creds creds;
krb5_get_init_creds_opt options;
if (krb5_init_context(&context) < 0)
return FALSE;
strlcpy(str, user, sizeof(str));
if (getcfg(lbs->name, "Kerberos Realm", realm, sizeof(realm))) {
strlcat(str, "@", sizeof(str));
strlcat(str, realm, sizeof(str));
}
if ((error = krb5_parse_name(context, str, &princ)) != 0) {
strlcpy(error_str, "<b>Kerberos error:</b> ", error_size);
strlcat(error_str, krb5_get_error_message(context, error), error_size);
strlcat(error_str, ". Please check your Kerberos configuration.", error_size);
return FALSE;
}
error = krb5_unparse_name(context, princ, &princ_name);
if (error) {
strlcpy(error_str, "<b>Kerberos error:</b> ", error_size);
strlcat(error_str, krb5_get_error_message(context, error), error_size);
strlcat(error_str, ". Please check your Kerberos configuration.", error_size);
return FALSE;
}
sprintf(str, "Using %s as server principal for authentication", princ_name);
write_logfile(lbs, str);
memset(&options, 0, sizeof(options));
krb5_get_init_creds_opt_init(&options);
memset(&creds, 0, sizeof(creds));
error = krb5_get_init_creds_password(context, &creds, princ,
(char *) password, NULL, NULL, 0, NULL, &options);
krb5_free_context(context);
if (error && error != KRB5KDC_ERR_PREAUTH_FAILED && error != KRB5KDC_ERR_C_PRINCIPAL_UNKNOWN) {
sprintf(error_str, "<b>Kerberos error %d:</b> ", error);
strlcat(error_str, krb5_get_error_message(context, error), error_size);
strlcat(error_str, ". Please check your Kerberos configuration.", error_size);
return FALSE;
}
if (error)
return FALSE;
return TRUE;
}
int auth_change_password_krb5(LOGBOOK * lbs, const char *user, const char *old_pwd, const char *new_pwd,
char *error_str, int error_size)
{
char *princ_name, str[256], realm[256];
int result_code, n;
krb5_error_code error;
krb5_data result_code_string, result_string;
krb5_principal princ;
krb5_context context;
krb5_creds creds;
krb5_get_init_creds_opt options;
if (krb5_init_context(&context) < 0)
return FALSE;
strlcpy(str, user, sizeof(str));
if (getcfg(lbs->name, "Kerberos Realm", realm, sizeof(realm))) {
strlcat(str, "@", sizeof(str));
strlcat(str, realm, sizeof(str));
}
if ((error = krb5_parse_name(context, str, &princ)) != 0) {
strlcpy(error_str, "<b>Kerberos error:</b> ", error_size);
strlcat(error_str, krb5_get_error_message(context, error), error_size);
strlcat(error_str, ". Please check your Kerberos configuration.", error_size);
return FALSE;
}
error = krb5_unparse_name(context, princ, &princ_name);
sprintf(str, "Using %s as server principal for authentication", princ_name);
write_logfile(lbs, str);
memset(&options, 0, sizeof(options));
krb5_get_init_creds_opt_init(&options);
krb5_get_init_creds_opt_set_tkt_life(&options, 300);
krb5_get_init_creds_opt_set_forwardable(&options, FALSE);
krb5_get_init_creds_opt_set_proxiable(&options, FALSE);
memset(&creds, 0, sizeof(creds));
error = krb5_get_init_creds_password(context, &creds, princ,
(char *) old_pwd, NULL, NULL, 0, "kadmin/changepw", &options);
if (error) {
strlcpy(error_str, "<b>Kerberos error:</b> ", error_size);
strlcat(error_str, krb5_get_error_message(context, error), error_size);
strlcat(error_str, ". Please check your Kerberos configuration.", error_size);
return FALSE;
}
error = krb5_set_password(context, &creds, (char *) new_pwd, princ,
&result_code, &result_code_string, &result_string);
if (error) {
strlcpy(error_str, "<b>Kerberos error:</b> ", error_size);
strlcat(error_str, krb5_get_error_message(context, error), error_size);
strlcat(error_str, ". Please check your Kerberos configuration.", error_size);
return FALSE;
}
if (result_code > 0) {
if (result_code_string.length > 0) {
strlcpy(error_str, result_code_string.data, error_size);
if ((int) result_code_string.length < error_size)
error_str[result_code_string.length] = 0;
}
if (result_string.length > 0) {
strlcat(error_str, ": ", error_size);
n = strlen(error_str) + result_string.length;
strlcat(error_str, result_string.data, error_size);
if (n < error_size)
error_str[n] = 0;
}
}
krb5_free_data_contents(context, &result_code_string);
krb5_free_data_contents(context, &result_string);
krb5_free_cred_contents(context, &creds);
krb5_get_init_creds_opt_free(context, &options);
krb5_free_context(context);
if (result_code > 0)
return FALSE;
return TRUE;
}
#endif
/*---- LDAP routines ------------------------------------------*/
#ifdef HAVE_LDAP
int ldap_init(LOGBOOK *lbs, char *error_str, int error_size)
{
char str[512], ldap_server[256];
int version;
int bind=0;
// Read Config file
if (getcfg(lbs->name, "LDAP server", ldap_server, sizeof(ldap_server))) {
strlcpy(str, ldap_server, sizeof(str));
}
else {
strlcpy(error_str, "<b>LDAP initialization error</b> ", error_size);
strlcat(error_str, " Please check your LDAP configuration.", error_size);
strlcat(str, "ERR: Cannot find LDAP server entry!", sizeof(str));
write_logfile(lbs, str);
return FALSE;
}
if (!getcfg(lbs->name, "LDAP userbase", ldap_userbase, sizeof(ldap_userbase))) {
strlcpy(error_str, "<b>LDAP initialization error</b> ", error_size);
strlcat(error_str, " Please check your LDAP configuration.", error_size);
strlcat(str, ", ERR: Cannot find LDAP userbase (e.g. \'ou=People,dc=example,dc=org\')!", sizeof(str));
write_logfile(lbs, str);
return FALSE;
}
if (!getcfg(lbs->name, "LDAP login attribute", ldap_login_attr, sizeof(ldap_login_attr))) {
strlcpy(error_str, "<b>LDAP initialization error</b> ", error_size);
strlcat(error_str, " Please check your LDAP configuration.", error_size);
strlcat(str, ", ERR: Cannot find LDAP login attribute (e.g. uid, cn, ...)!", sizeof(str));
write_logfile(lbs, str);
return FALSE;
}
if (!getcfg(lbs->name, "LDAP DN User", ldap_dn_user, sizeof(ldap_dn_user))) {
strlcpy(error_str, "<b>LDAP initialization error</b> ", error_size);
strlcat(error_str, " Please check your LDAP configuration.", error_size);
strlcat(str, ", ERR: Cannot find LDAP login attribute (e.g. uid, cn, ...)!", sizeof(str));
write_logfile(lbs, str);
return FALSE;
}
if (!getcfg(lbs->name, "LDAP PW User", ldap_pw_user, sizeof(ldap_pw_user))) {
strlcpy(error_str, "<b>LDAP initialization error</b> ", error_size);
strlcat(error_str, " Please check your LDAP configuration.", error_size);
strlcat(str, ", ERR: Cannot find LDAP login attribute (e.g. uid, cn, ...)!", sizeof(str));
write_logfile(lbs, str);
return FALSE;
}
// Initialize/open LDAP connection
if(ldap_initialize( &ldap_ld, ldap_server )) {
perror("ldap_initialize");
strlcpy(error_str, "<b>LDAP initialization error</b> ", error_size);
strlcat(error_str, " Please check your LDAP configuration.", error_size);
return FALSE;
}
// Use the LDAP_OPT_PROTOCOL_VERSION session preference to specify that the client is LDAPv3 client
version = LDAP_VERSION3;
ldap_set_option(ldap_ld, LDAP_OPT_PROTOCOL_VERSION, &version);
write_logfile(lbs, str);
return TRUE;
}
int auth_verify_password_ldap(LOGBOOK *lbs, const char *user, const char *password, char *error_str,
int error_size)
{ LDAPMessage *result, *err, *entry;
int bind=0, i, rc=0, nb=0;
char str[512], filter[512];
char *attribute , *dn;
BerElement *ber;
BerValue **values;
ldap_ld = NULL;
memset(&ldap_bindDN[0], 0, sizeof(ldap_bindDN));
struct timeval timeOut = {3,0}; // 3 second connection/search timeout
// zerotime.tv_sec = zerotime.tv_usec = 0L;
if(!ldap_init(lbs,error_str,error_size)) {
strlcpy(error_str, "<b>LDAP initialization error</b> ", error_size);
strlcat(error_str, " Please check your LDAP configuration.", error_size);
return FALSE;
}
printf("\n dn: %s\n", ldap_dn_user );
//Bind with read account
bind = ldap_simple_bind_s(ldap_ld, ldap_dn_user, ldap_pw_user, LDAP_AUTH_SIMPLE);
if(bind != LDAP_SUCCESS) {
strlcpy(error_str, "<b>LDAP BIND error with read account</b> ", error_size);
strlcat(error_str, " Please check your LDAP configuration.", error_size);
return FALSE;
}
// search user
sprintf(filter, "(%s=%s)", ldap_login_attr, user);
rc = ldap_search_ext_s(
ldap_ld, // LDAP session handle
ldap_userbase, // Search Base
... 318 more lines ...
|
149
|
Sat Jun 15 06:13:07 2019 |
John | secondcomingtechnologies@fastmail.com | Script | Re: Custom input forms implementation | Stable | Sat Jun 15 06:19:24 2019 by John | I have been trying to get my head around this application module. I assume that after the input is done on this example (ShiftCheck), if goes into the usual file system directorys for storage? Or is it (or can it) goto another db like sql, flat, etc.? I also assume that the 'normal' Elog screens we see for input (and output), would be a completely seperate module that you have for (ShiftCheck).. but we do not see them here (as one of the attachments)? I am asking these questions because I am trying to recreate this (type) of input/output system for users, so I would like to know how the 'whole picture' is done with your example here. Thanx again.
Stefan Ritt wrote: |
Dear ELOG users,
starting with SVN revision 2328, custom input forms are implemented. This allows application specific formats for check lists etc. In our specific case we had to implement a shift check list, which was quite long. Furthermore the check list should be optimized for an iPad, which we take in the field and record various checks and readings (in our case some gas pressure gauges at the PSI particle accelerator). Since the standard ELOG interface was too inflexible, a completely hand-written form was needed. The form can be activated by the new configuration options Custom New Form, Custom Edit Form and Custom Display Form, one for a new entry, an entry to edit and and entry to display. In our case we used the same form for all three cases. This is how the shift check list looks under the Safari Browser on a PC:
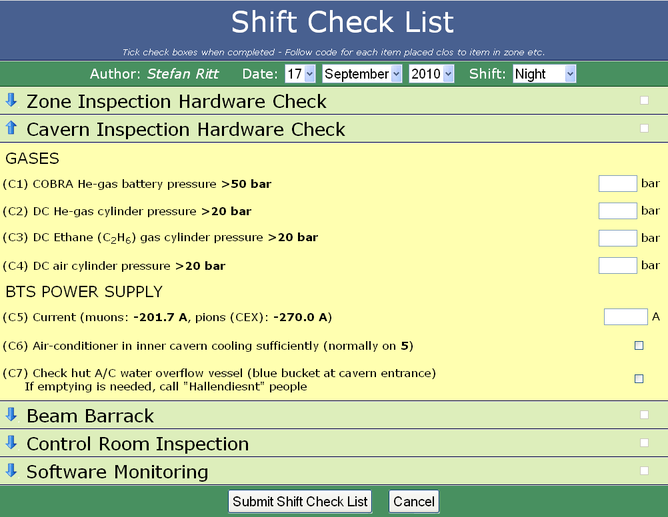
And here is how it looks on the iPad:
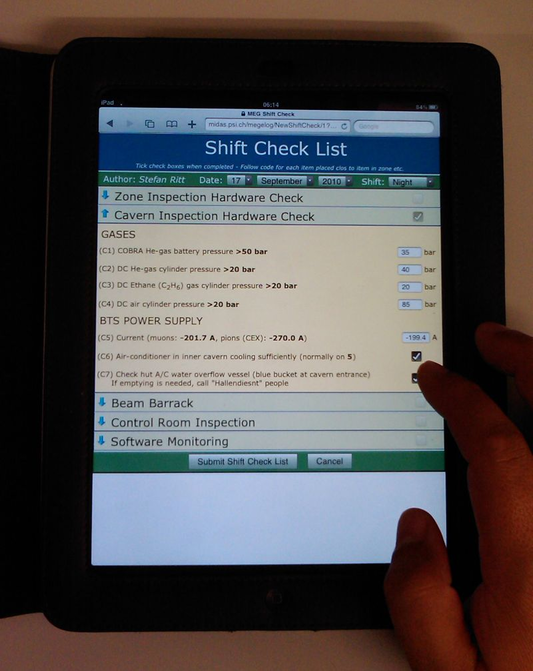
Each section can be collapsed and expanded (blue arrows at the left), and various internal checks are made before the check list can be submitted.
Implementing such forms is however more something for the advanced user, since you have to hand-write HTML with CSS and JavaScript code. It can then however be a powerful method for check lists. Please find in the attachments the elogd.cfg configuration for that logbook and the shiftcheck.html source code file. It is a bit complicated since the page is a static page, elogd just serves it from the file. This requires all the dynamic functions to be implemented inside the HTML file with JavaScript. To display an entry for example, the JavaScript loads the raw data with the "?cmd=Download" command and the populates the form fields. The collapsing and expanding is done by using CSS properties. The integrated style sheet was optimized for the rendering on an iPad. Rather large fonts were chosen so that the items can be checked easily with your finger tips. Various parameters are sent between the browser and the elogd program via hidden fields and cookies. So only something for experts! But if you go through the effort and hand-write the form, it can be very handy. Note that you have to upgrade to SVN revision 2328 for the three new options.
|
|
11
|
Wed Nov 24 23:45:19 2004 |
damon nettles | nettles@phgrav.phys.lsu.edu | Other | Steps for securing Elog using SSL and Apache | Stable | | Everything in this guide was done on a full install of Fedora Core 3 running
Apache 2.0. If you are using an older version of Apache some of this may not
work, so I recommend upgrading. Also, on different Linux distributions, some
of the paths may be different.
The goal here is to get Elog set up under Secure Socket Layers, so that
communication both ways is encrypted. This will cover any password
transactions so nothing gets sent over the web in the clear.
The previous method of securing the Elog, which involved using stunnel, is
out of date. A better way to go is to use the Elog in conjunction with
Apache. The Apache method leverages all the research and development that's
gone into providing secure sockets for Apache, and removes the need for any
serious reinventing of the wheel.
We begin with a web server running on port 80 and an Elog server running on
port 8080.
Making Certificates:
It's necessary to generate some secure certificates to be issued to anyone
who attempts to access the securesite.
A guide to making the certificates can be found at:
http://slacksite.com/apache/certificate.html
So, following the steps in the article:
openssl genrsa -des3 -rand file1:file2:file3:file4:file5 -out\
server.key 1024
where the \ is merely an indicator that the command wouldn't fit on a line
here. The fileN references are sources of random information to help the
random number seed be more random. I merely used some personal text files
that were zipped up, as suggested in the page.
openssl rsa -in server.key -out server.pem
Removes the RSA encryption from the key, to make it easier for the Apache
server to deal with it.
openssl req -new -key server.key -out server.csr
Starts a line of questioning about us as a certificate issuing entity.
Answer with reasonable values.
openssl x509 -req -days 60 -in server.csr -signkey server.key -
out\
server.crt
After this move the server.pem, server.crt, and server.csr to the
appropriate directories under /etc/httpd/conf/ . The extensions explain
which directory to put them in, with the exception that server.pem ended up
in etc/httpd/conf/ssl.key/ .
In the elogd.cfg file, change the port to 8079, and set the URL to
"https://your.host.name/" . Restarting the Elog daemon now leaves us with
Elog listening to port 8079 instead of port 8080.
The rest of the story is in the "elogredirect.conf" file attached to this
post, but here are the highlights.
Create a virtual host dealing with SSL that listens to port 443 (the ssl
port), and acts as a proxy for port 8079 (where Elog is listening). This
allows Apache to act as an SSL handler for Elog by handing off any access at
https://your.host.name/ to the Elog server. The firewall then can keep out
any direct attempts to access port 8079, so that the only thing that can
reach the Elog server is stuff talking to 8079 on the local side of the
firewall (which pretty much means just the Apache proxy). I recommend
Firestarter for the firewall config by the way, it's a real lifesaver.
http://firestarter.sourceforge.net/
This covers the SSL portion of the story, and by doing the redirection
inside the port 443 virtual host, instead of from the port 80 webpage as
before, you can avoid any path overlap.
As was the case for us, you may have links in older Elog posts, e-mails, or
web pages that point to specific Elog posts. If you have been using Elog for
some time and never bothered with the SSL stuff, the links most likely look
something like
http://your.host.name:8080/yourlogbook/postnumber.
To cover legacy support for calls on port 8080, you can create another
virtual host listening to port 8080. This host's job is to take any
incoming URL calls on "http://your.host.name:8080/a_directory" and
translate them into calls on "https://your.host.name/the_same_directory" .
This means that any attempt to contact the Elog on port 8080 will get
answered by an Apache virtual host that redirects the client through the
Apache SSL virtual host described above. See the conf file for the details.
So in the end, the firewall is set to only allow through ports 80, 443, and
8080. Port 80 handles the normal webpage access stuff. Port 443
exclusively handles the SSL port for the Elog daemon, and port 8080
exclusively handles the redirect for the legacy Elog calls.
Implementation of this setup on another system should be pretty
straightforward. Apache's config file is at /etc/httpd/conf/httpd.conf ,
and it also loads any *.conf files in /etc/httpd/conf.d/ . So its a pretty
simple case of just dropping elogredirect.conf into /etc/httpd/conf.d/ and
restarting the Apache server. Of course the necessary changes to elogd.cfg
have to be made and that server restarted as well. The firewall, too, needs
to be setup to secure the whole deal. Note that the elogredirect.conf file
needs to be edited for your specific setup (changing the instances of
"your.host.name" to whatever your server is, and also putting in the
administrator e-mail address where it is noted).
This work was done by Jonathan Hanson and Damon Nettles in the Gravity Lab
at Louisiana State University. You can see our Elog at
https://sam.phys.lsu.edu/elog .
If you have any questions or comments send them to
nettles@phgrav.phys.lsu.edu . |
Attachment 1: elogredirect.conf
|
### Here be things to make the elogd daemon invisibly secure under an
### Apache SSL proxy virtual host. Arrrrrr!
### ----This config file be mostly written by Jonathan Hanson, 11/23/04
### ----With some help from a few old salts on the net.
### This be the first instance of SSL in our setup, so the SSL module
### Must be called. This can be commented out if it don't put wind in
### your sails.
LoadModule ssl_module modules/mod_ssl.so
### Ahoy, ye scurvy land dogs! Listen to the SSL port (443) or may the
### sea beasts take ye!
Listen 443
### Make a virtual host at the default server name, and assign it port 443.
<VirtualHost _default_:443>
### Here be standard configuration for the Virtual Host
ServerAdmin your_e-mail_address
ServerName _default_:443
RequestHeader set Front-End-Https "On"
### This be the path to the elog directory
### (This didn't seem to make any difference, but it be a good
### idea nonetheless)
DocumentRoot /usr/local/elog
### Here be the setup for the SSL component of the Virtual Host
SSLEngine On
SSLCertificateFile /etc/httpd/conf/ssl.crt/server.crt
SSLCertificateKeyFile /etc/httpd/conf/ssl.key/server.pem
### Here be the setup options for the Proxy module
ProxyRequests Off
ProxyPreserveHost On
### This be the root of the new Virtual Host, and it should be
### redirected to the port the elogd server is listening to
### (8079 on our poop deck).
<Location />
ProxyPass http://your.host.name:8079/
ProxyPassReverse http://your.host.name:8079/
SSLRequireSSL
</Location>
### Shiver me timbers! A firewall can be laid across the elog
### port to hinder direct access from the outside world to the elog
### daemon. This'll make the scurvy wretches come in through the apache
### proxy virtual host, and batten down the hatches on the elog in general.
</VirtualHost>
### Our previous elog configuration was at http://your.host.name:8080 and some of our
### users made static HTML links to other posts in their posts and email. They be sleeping
### in Davy Jones's locker in the briney deep now, but we be needing to make these posts
### backward-compatible. We be changing the port Elogd listens to (as above) to 8079, and
### then we be using another new virtual host at port 8080 to redirect to the new SSL URL.
### So it appears to the landlubbers outside as if a normal elog server is listening to port
### 8080, but in reality it be a Virtual Host redirecting through the other SSL virtual host
### which then be passing it on to the port the elog server really be listening to.
### If ye not be needing this backwards compatability, the following section can be made
### to walk the plank.
### I won't be telling ye twice, ye slimy bilge rat! Pay attention to what
### used to be the old elog port(8080).
Listen 8080
<VirtualHost _default_:8080>
### Here be standard configuration for the Virtual Host
ServerAdmin your_e-mail_address
ServerName _default_:8080
### This be the path to the html directory
### (This didn't seem to make any difference, but it be a good
### idea nonetheless)
DocumentRoot /httpd/html
### Here be the dark magic of mod_rewrite. Quake in your boots ye dogs!
<IfModule mod_rewrite.c>
RewriteEngine On
RewriteRule ^/(.*) https://your.host.name/$1 [NC,R=301,L]
</IfModule>
</VirtualHost>
### In the end, elog be reachable through either https://your.host.name/
### or http://your.host.name:8080/ , though the latter will be rewritten
### to the former as soon as the request be made. This be satisfying our
### needs for backwards compatbility with old URLs, while ensuring modern
### secure SSL support. Beware matey, recognize that we also had to change
### the port that elog listens to, and then add a URL line in the elogd.cfg
### file:
### port = 8079
### URL = https://your.host.name/
### Also a firewall was brought up and told to allow through only ports
### 80(html), 443(SSL), and 8080(the elog stand-in) and of course any other
### ports ye may need for other applications.
|
15
|
Thu Jul 28 18:42:48 2005 |
Emiliano Gabrielli | AlberT@SuperAlberT.it | Other | [New Feature]: JS calendar filter | Beta | Thu Aug 4 11:20:36 2005 by Emiliano Gabrielli | NOTE:
it seems that for some strange reason it slows down elog very much when and only when elog is stunneled over ssl!
The attached tarball contains a full featured JavaScript allowing everyone to use a JS calendar (no popup windows, just JS and CSS!) to perform date-based filtering actions. An uncompressed version and the tarball for the last cvs rvision of this script is available at www.SuperAlberT.it
You can browse the ChangeLog here.
A simple way to use it is to uncompress the wall package into the elog data dir, under the "scripts" subdir.
the you can add the following code to your elog.cfg:
Filter Menu text = scripts/calendar_filter/calendar_filter.html
note that this configuration parameter is available in elog starting from
Revision 1.732 2005/07/29
and automagically have the new calendar_filter icon showed 
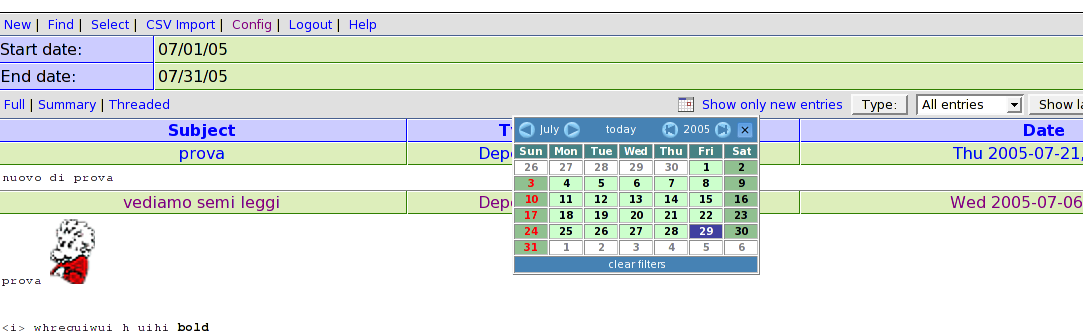 |
Attachment 2: elog_calendar_filter-1.0.1.tar.gz
|
16
|
Wed Sep 7 16:52:30 2005 |
Peter Eriksson | peter@ifm.liu.se | Other | Solaris 10 SMF/Greenline management manifest for ELog | Stable | | Please find enclosed as an attachment a Solaris 10 SMF/Greenline manifest that can be used to manage ELog.
(If you don't know what it is - it replaces init.d/cron/inittab and more stuff) |
Attachment 1: elog.xml
|
<?xml version="1.0"?>
<!DOCTYPE service_bundle SYSTEM "/usr/share/lib/xml/dtd/service_bundle.dtd.1">
<!--
Copyright (c) 2005-06-17 Peter Eriksson (peter@ifm.liu.se)
This manifest can be used to manage an elog daemon using the
Solaris SMF subsystem.
Import this manifest using:
svccfg -v import elog.xml
Then activate the daemon with:
svcadm enable site/elog
-->
<service_bundle type='manifest' name='IFM:elog'>
<service
name='site/elog'
type='service'
version='1'>
<single_instance />
<!-- Need / & /usr filesystems mounted, /var mounted read/write -->
<dependency
name='fs-local'
type='service'
grouping='require_all'
restart_on='none'>
<service_fmri value='svc:/system/filesystem/local' />
</dependency>
<dependency
name='network-service'
grouping='require_all'
restart_on='none'
type='service'>
<service_fmri value='svc:/network/service' />
</dependency>
<dependency
name='name-services'
grouping='require_all'
restart_on='refresh'
type='service'>
<service_fmri value='svc:/milestone/name-services' />
</dependency>
<exec_method
type='method'
name='start'
exec='/ifm/sbin/elogd -D -c /ifm/etc/elogd.cfg'
timeout_seconds='60'>
</exec_method>
<exec_method
type='method'
name='stop'
exec=':kill'
timeout_seconds='30'>
</exec_method>
<instance name='default' enabled='false' />
<stability value='Evolving' />
<template>
<common_name>
<loctext xml:lang='C'>
Electronic Logbook server
</loctext>
</common_name>
<documentation>
<manpage title='elogd' section='1' manpath='/usr/local/man' />
</documentation>
</template>
</service>
</service_bundle>
|
22
|
Wed Jul 11 11:13:16 2007 |
Peter Rienstra | peter.rienstra@gmail.com | Other | Compiling elogd.c on HP-UX 64 bit | Beta | Thu Jul 12 09:38:47 2007 by Peter Rienstra | We succeeded in compiling and running elogd (elog-2.6.5) on HP-UX 64 bit Itanium platform (HP-UX B.11.23 U ia64).
The main problem was we got a core dump after starting elogd. The cause was that the memory has be allocated with a 4 byte boundary. This could be the case on other 64 bit platforms as well. A colleague of mine (Sander Notting) found the solution.
Unzip and untar the zip file (elog-latest.tar.gz)
Go to the src directory (elog-2.6.5/src)
Edit elogd.c
Replace all:
show_selection_page(NULL); => show_selection_page();
seteuid => setuid
setegid => setgid
On line 564:
void *buffer => char *buffer
Line 645, add the text in bold:
void *xmalloc(size_t bytes)
{
char *temp;
/* Align buffer on 4 byte boundery for HP UX and other 64 bit systems to prevent Bus error(core dump)*/
if (bytes & 3)
bytes += 4 - (bytes & 3);
temp = (char *) malloc(bytes + 12);
After that compile:
cc -w -c -o regex.o regex.c
cc -w -c -o mxml.o ../../mxml/mxml.c
cc -w -c -o strlcpy.o ../../mxml/strlcpy.c
cc -I../../mxml -o elogd elogd.c regex.o mxml.o strlcpy.o
We didn't try to run elogd under root yet. |
Attachment 1: elogdhpux64.c.gz
|
23
|
Fri Jul 13 12:36:45 2007 |
Stefan Ritt | stefan.ritt@psi.ch | Other | Re: Compiling elogd.c on HP-UX 64 bit | Beta | Thu Jul 12 09:38:47 2007 by Peter Rienstra | I applied most of your patches to the elog source code, SVN revision 1885. The only missing piece has to do with seteuid/setuid. I definitively need seteuid for linux, because elogd might be started under root, then it falls back to an optional elog user. But when it stops, it has to restore the original root user in order to delete the PID file (/var/run/elogd.pid) which was created under root. If seteuid does not exist under HP-UX, you should add something like
#ifdef HP-UX
setuid(...)
#else
seteuid(...)
#endif
Probably the HP-UX has to be something else, but I cannot test this since I don't have such an OS here. Once you get this working I can put it into the standard distribution. |
24
|
Mon Jul 16 15:27:08 2007 |
Peter Rienstra | peter.rienstra@gmail.com | Other | Re: Compiling elogd.c on HP-UX 64 bit | Beta | Thu Jul 12 09:38:47 2007 by Peter Rienstra | Stefan,
First I want to say I really like your program. We work in a small group of 5 database administrators, and this is exactly what we need to inform each other. Elog is simple but very functional, so thanks!
My problem is that I don't have root access to the HP-UX machines. We don't run elogd as root, so I wasn't really interested in the seteuid functionality, I just wanted to compile and run the program.
HP-UX doesn't have the "seteuid" and "setegid" functions. But there are "setuid+setgid", "setreuid+setregid" and "setresuid+setresgid" functions available. I'm not sure which one is the best to use. I uploaded the manpages as attachment. I hope this will help you.
If you want I can do a compile and run test on HP-UX with your altered source code. But I can't do a test with "root".
Stefan Ritt wrote: | I applied most of your patches to the elog source code, SVN revision 1885. The only missing piece has to do with seteuid/setuid. I definitively need seteuid for linux, because elogd might be started under root, then it falls back to an optional elog user. But when it stops, it has to restore the original root user in order to delete the PID file (/var/run/elogd.pid) which was created under root. If seteuid does not exist under HP-UX, you should add something like
#ifdef HP-UX
setuid(...)
#else
seteuid(...)
#endif
Probably the HP-UX has to be something else, but I cannot test this since I don't have such an OS here. Once you get this working I can put it into the standard distribution. |
|
Attachment 1: man_setuid.txt
|
setuid(2) setuid(2)
NAME
setuid(), setgid() - set user and group IDs
SYNOPSIS
#include <unistd.h>
int setuid(uid_t uid);
int setgid(gid_t gid);
DESCRIPTION
setuid() sets the real-user-ID (ruid), effective-user-ID (euid),
and/or saved-user-ID (suid) of the calling process. If the Security
Containment product is installed, these interfaces treat a process
observing CHSUBJIDENT as a privileged process. Otherwise, only
processes with an euid of zero are treated as privileged processes.
See privileges(5) for more information on Security Containment and
fine-grained privileges.
The following conditions govern setuid's behavior:
+ If the process is privileged, setuid() sets the ruid, euid,
and suid to uid.
+ If the process is not privileged and the argument uid is equal
to the ruid or the suid, setuid() sets the euid to uid; the
ruid and suid remain unchanged. (If a set-user-ID program is
not running as superuser, it can change its euid to match its
ruid and reset itself to the previous euid value.)
+ If the process is not privileged, the argument uid is equal to
the euid, and the calling process has the PRIV_SETRUGID
privilege, setuid() sets the ruid to uid; the euid and suid
remain unchanged.
setgid() sets the real-group-ID (rgid), effective-group-ID (egid),
and/or saved-group-ID (sgid) of the calling process. The following
conditions govern setgid()'s behavior:
+ If the process is privileged, setgid() sets the rgid and egid
to gid.
+ If the process is not privileged and the argument gid is equal
to the rgid or the sgid, setgid() sets the egid to gid; the
rgid and sgid remain unchanged.
+ If the process is not privileged, the argument gid is equal to
the egid, and the calling process has the PRIV_SETRUGID
privilege, setgid() sets the rgid to gid; the egid and sgid
remain unchanged.
Hewlett-Packard Company - 1 - HP-UX 11i Version 2: May 2006
setuid(2) setuid(2)
Security Restrictions
Some or all of the actions associated with this system call require
the CHSUBJIDENT privilege. Processes owned by the superuser have this
privilege. Processes owned by other users may have this privilege,
depending on system configuration.
See privileges(5) for more information about privileged access on
systems that support fine-grained privileges.
RETURN VALUE
Upon successful completion, setuid() and setgid() return 0; otherwise,
they return -1 and set errno to indicate the error.
ERRORS
setuid() and setgid() fail and return -1 if any of the following
conditions are encountered:
[EPERM] None of the conditions above are met.
[EINVAL] uid (gid) is not a valid user (group) ID.
WARNINGS
It is recommended that the PRIV_SETRUGID capability be avoided, as it
is provided for backward compatibility. This feature may be modified
or dropped from future HP-UX releases. When changing the real user ID
and real group ID, use of setresuid() and setresgid() (see
setresuid(2)) is recommended instead.
AUTHOR
setuid() was developed by AT&T, the University of California,
Berkeley, and HP.
setgid() was developed by AT&T.
SEE ALSO
exec(2), getuid(2), setresuid(2), privileges(5).
STANDARDS CONFORMANCE
setuid(): AES, SVID2, SVID3, XPG2, XPG3, XPG4, FIPS 151-2, POSIX.1
setgid(): AES, SVID2, SVID3, XPG2, XPG3, XPG4, FIPS 151-2, POSIX.1
Hewlett-Packard Company - 2 - HP-UX 11i Version 2: May 2006
|
Attachment 2: man_setreuid.txt
|
setreuid(2) setreuid(2)
NAME
setreuid - set real and effective user IDs
SYNOPSIS
#include <unistd.h>
int setreuid(uid_t ruid, uid_t euid);
DESCRIPTION
The setreuid() function sets the real and effective user IDs of the
current process to the values specified by the ruid and euid
arguments. If ruid or euid is -1, the corresponding effective or real
user ID of the current process is left unchanged.
A process with appropriate privileges can set either ID to any value.
An unprivileged process can only set the effective user ID if the euid
argument is equal to either the real, effective, or saved user ID of
the process.
It is unspecified whether a process without appropriate privileges is
permitted to change the real user ID to match the current real,
effective or saved user ID of the process.
RETURN VALUE
Upon successful completion, 0 is returned. Otherwise, -1 is returned
and errno is set to indicate the error.
ERRORS
The setreuid() function will fail if:
[EINVAL] The value of the ruid or euid argument
is invalid or out-of-range.
[EPERM] The current process does not have
appropriate privileges, and either an
attempt was made to change the effective
user ID to a value other than the real
user ID or the saved set-user-ID or an
attempt was made to change the real user
ID to a value not permitted by the
implementation.
SEE ALSO
getuid(2), setuid(2), <unistd.h>.
CHANGE HISTORY
First released in Issue 4, Version 2.
Hewlett-Packard Company - 1 - HP-UX 11i Version 2: August 2003
|
Attachment 3: man_setresuid.txt
|
setresuid(2) setresuid(2)
NAME
setresuid, setresgid - set real, effective, and saved user and group
IDs
SYNOPSIS
#include <unistd.h>
int setresuid(uid_t ruid, uid_t euid, uid_t suid);
int setresgid(gid_t rgid, gid_t egid, gid_t sgid);
DESCRIPTION
setresuid() sets the real, effective and/or saved user ID of the
calling process.
If the current real, effective or saved user ID is equal to that of a
user having appropriate privileges, setresuid() sets the real,
effective and saved user IDs to ruid, euid, and suid, respectively.
Otherwise, setresuid() only sets the real, effective, and saved user
IDs if ruid, euid, and suid each match at least one of the current
real, effective, or saved user IDs.
If ruid, euid, or suid is -1, setresuid() leaves the current real,
effective or saved user ID unchanged.
setresgid() sets the real, effective and/or saved group ID of the
calling process.
If the current real, effective or saved user ID is equal to that of a
user having appropriate privileges, setresgid() sets the real,
effective, and saved group ID to rgid, egid, and sgid, respectively.
Otherwise, setresgid() only sets the real, effective and saved group
ID if rgid, egid, and sgid each match at least one of the current
real, effective or saved group ID.
If rgid, egid, or sgid is -1, setresgid() leaves the current real,
effective or saved group ID unchanged.
Security Restrictions
Some or all of the actions associated with this system call require
the PRIV_CHSUBJIDENT privilege (CHSUBJIDENT). Processes owned by the
superuser will have this privilege. Processes owned by other users
may have this privilege, depending on system configuration. See
privileges(5) for more information about privileged access on systems
that support fine-grained privileges.
RETURN VALUE
Upon successful completion, setresuid() and setresgid() return 0;
otherwise, they return -1 and set errno to indicate the error.
Hewlett-Packard Company - 1 - HP-UX 11i Version 2: May 2005
setresuid(2) setresuid(2)
ERRORS
setresuid() and setresgid() fail if any of the following conditions
are encountered:
[EINVAL] ruid, euid, or suid (rgid, egid, or sgid) is not a
valid user (group) ID.
[EPERM] None of the conditions above are met.
AUTHOR
setresuid() and setresgid() were developed by HP.
SEE ALSO
exec(2), getuid(2), setuid(2).
Hewlett-Packard Company - 2 - HP-UX 11i Version 2: May 2005
|
25
|
Mon Jul 16 15:57:47 2007 |
Stefan Ritt | stefan.ritt@psi.ch | Other | Re: Compiling elogd.c on HP-UX 64 bit | Beta | Thu Jul 12 09:38:47 2007 by Peter Rienstra |
Peter Rienstra wrote: | HP-UX doesn't have the "seteuid" and "setegid" functions. But there are "setuid+setgid", "setreuid+setregid" and "setresuid+setresgid" functions available. |
I had a look and found that setreuid/setregid is also available under Linux, so I use those functions instead, which should also work on HP-UX. Can you check revision 1888 (http://savannah.psi.ch/viewcvs/trunk/src/elogd.c?root=elog&rev=1888), compile it and see if you can run it at least under your non-root account. |
26
|
Mon Jul 16 16:43:07 2007 |
Peter Rienstra | peter.rienstra@gmail.com | Other | Re: Compiling elogd.c on HP-UX 64 bit | Beta | Thu Jul 12 09:38:47 2007 by Peter Rienstra |
I downloaded revision 1888. There were no problems compiling it. It's running on the HP-UX system now and everything seems to work fine.  |
|